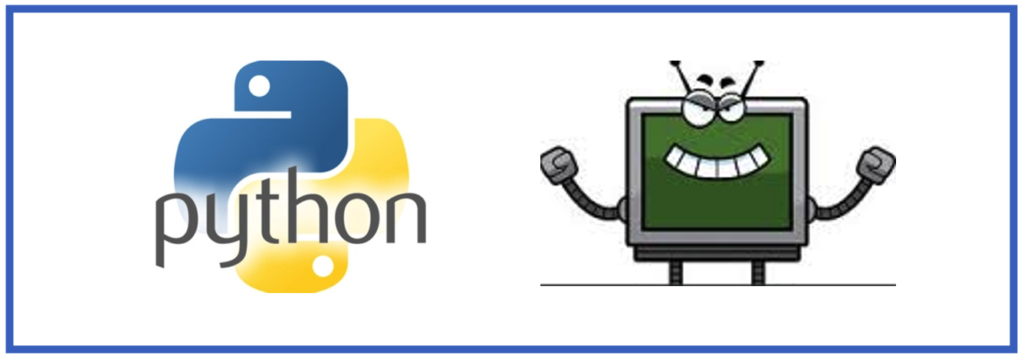
Introduction
One of the key characteristics and advantage of a computer science background is an acute ability to analyze data and utilize the power of modern computers. We have already explored manipulating and visualizing data in Excel; in today’s lab you will learn to create simple programs in Python, and eventually get a feel for the power and freedom this allows you for analyzing large sets of data.
Python is a widely used general-purpose, high-level programming language that allows you to tap into the power of modern computers. Python uses a set of commands to manipulate data and create tools, with a design philosophy that emphasizes code readability. Its syntax allows programmers to express concepts in fewer lines of code than would be possible in languages such as C++ or Java.
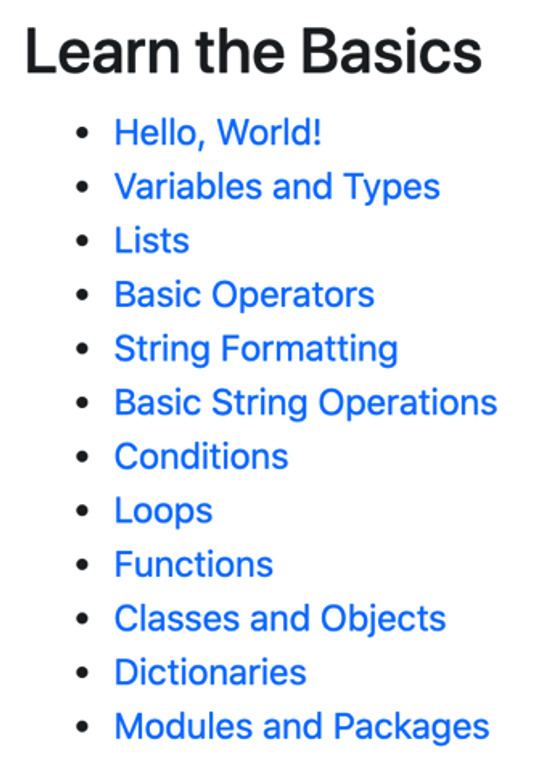
When programming, remember that the computer cannot interpret anything other than the code you enter into the program. All commands will be followed exactly, so you must tell the computer very specifically – and correctly – what to do. Python can be downloaded for free at https://www.python.org/
Today, we will work through an online tutorial that will allow you to learn Python codes without needing the program to be installed on your computer.
In the tables in your worksheet, you will type in the code that you use to run the listed operation, or answer questions about the activities you are performing.
Hello World
Access the tutorial here: https://www.learnpython.org/en/Hello,_World!
(The link is behaving strangely, so just copy and paste this test into your browser window).
- Print a string that reads “This line will be printed.”
- Print a string with a favorite quote.
- What is the message you receive when you do NOT include the correct amount of indentation spaces?
- Print command to print the line, “Hello, World!”
Variables and Types
Numbers
- What is the difference between an integer, and a floating point number?
- Type the two lines of code that will return the number “8”
- Which of the two options for defining a floating point number seems easiest for you to remember?
Strings
- What happens when you attempt to print the following code (copy and paste it into the tutorial):
- mystring = ‘It’s the ship that made the Kessel run in less than twelve parsecs.’
- print(mystring)
- How would you fix this code? Type the code that will not return that error:
- In the window that begins: a, b = 3, 4
- Write a line of code here that would return the number 7.
- Write a line of code that would return the number 14.
- Write a line of code that would return the number 12.
- Complete the Exercise at the bottom of this page, and type in your changes to the three lines of code below:
- mystring = None
- myfloat = None
- myint = None
Lists
An array is a data structure that stores values of same data type. In Python, this is the main difference between arrays and lists. While python lists can contain values corresponding to different data types, arrays in python can only contain values corresponding to same data type.
- In the lines of code below, explain why running print(mylist[2]) will return the number 3.
- mylist = []
- mylist.append(A)
- mylist.append(B)
- mylist.append(C)
- print(mylist[0]) # prints 1
- print(mylist[1]) # prints 2
- print(mylist[2]) # prints 3
- Fix the code below to return the number 1
- mylist = [1,2,3]
- print(mylist[10])
- Type in the code you used to correctly complete this exercise.
Basic Operations
Quick intro to order of operations:
You may be familiar with PEMDAS:
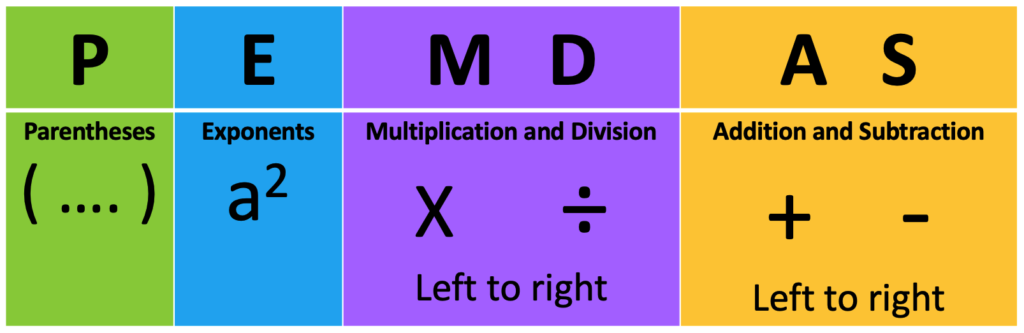
- Although we haven’t yet covered order of operations in this class, after referring to the information above, can you determine which of the following best describes the way that Python calculated the equation in the first box?
A | B | C |
1 + 2 = 3 3 / 4.0 = .75 3 / .75 | 1 + 2 = 3 3 * 3 = 9 9 / 4.0 | 2 * 3 = 6 6 / 4.0 = 1.5 1.5 + 1 |
- Does Python use order of operations?
- Predict what the answer will be to the following operation:
- number = 3 * 4 / 1 + 2
- print(number)
- Now paste this formula into the tutorial. Was your guess correct? Which of the following best describes the way Python calculated this number?
A | B | C |
3 * 4 = 12 12 / 1 = 12 12 + 2 | 3 * 4 = 12 1 + 2 = 3 12 / 3 | 1 + 2 = 3 4 / 3 = 1.33 1.33 * 3 |
- Type in the code to multiply 42 by 83
- Paste in the code to complete this exercise.
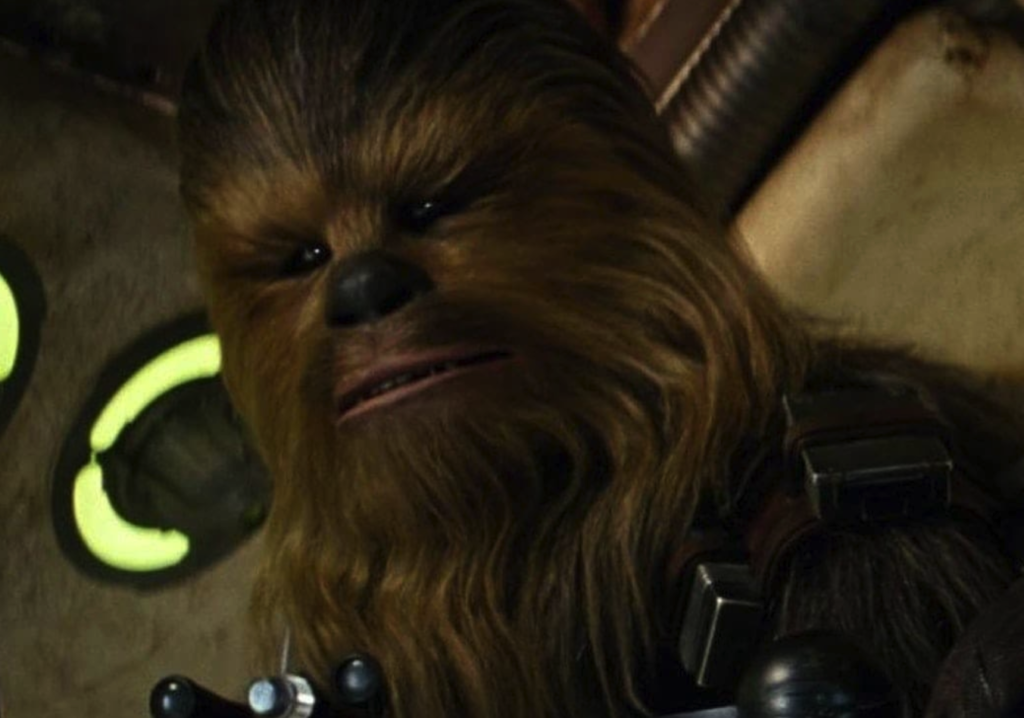
String Formatting
- Type in the code to print “Hello, Professor St. John”
- Type in the code to print “Chewbacca is 234 years old”
- Paste in the code to complete the exercise.
If you complete everything above before the end of class (and feel as though you understand all the material above), please continue to work through the rest of the worksheet, as time permits.
Basic String Operations
- Type in the code to count how many times the letter “e” appears in “The Empire Strikes Back”
- NOTE: the code that includes “afewwords” to split the string does not appear to work as written on the site. Try this instead:
- astring = “Hello world!”
- print(“Split the words of astring: %s” % astring.split(” “))
- How would we be able to do a similar function in Excel?
- Paste in the code to complete the exercise.
Conditions
- What does a Boolean operator do?
- Type in the code that would return “Your name is Luke, and you are 19 years old”
- Type in the code that would return “Darth Vader is the father of either Luke or Han Solo.”
- Complete the exercise.
Loops
- Complete the exercise.
Python Glossary
Adapted from https://www.pythonforbeginners.com/python-glossary
- “>>>”: The default Python prompt of the interactive shell. Often seen for code examples which can be executed interactively in the interpreter.
- abs: Return the absolute value of a number.
- argument: Extra information which the computer uses to perform commands.
- array: a data structure that stores values of same data type.
- assert: Used during debugging to check for conditions that ought to apply
- assignment: Giving a value to a variable.
- block: Section of code which is grouped together
- break: Used to exit a for loop or a while loop.
- class: A template for creating user-defined objects.
- compiler: Translates a program written in a high-level language into a low-level language.
- continue: Used to skip the current block, and return to the “for” or “while” statement
- conditional statement: Statement that contains an “if” or “if/else”.
- debugging: The process of finding and removing programming errors.
- def: Defines a function or method
- dictionary: A mutable associative array (or dictionary) of key and value pairs. Can contain mixed types (keys and values). Keys must be a hashable type.
- docstring: A docstring is a string literal that occurs as the first statement in a module, function, class, or method definition.
- evaluation order: Python evaluates expressions from left to right. Notice that while evaluating an assignment, the right-hand side is evaluated before the left-hand side.
- exceptions: Means of breaking out of the normal flow of control of a code block in order to handle errors or other exceptional conditions
- expression: Python code that produces a value.
- filter: filter(function, sequence) returns a sequence consisting of those items from the sequence for which function(item) is true
- float: An immutable floating point number.
- for: Iterates over an iterable object, capturing each element to a local variable for use by the attached block
- function: A parameterized sequence of statements.
- function call: An invocation of the function with arguments.
- garbage collection: The process of freeing memory when it is not used anymore.
- generators: A function which returns an iterator.
- high level language: Designed to be easy for humans to read and write.
- IDLE: Integrated development environment
- if statement: Conditionally executes a block of code, along with else and elif (a contraction of else-if).
- immutable: Cannot be changed after its created.
- import: Used to import modules whose functions or variables can be used in the current program. Brings in a library of tools and available commands. Every library hold lots of new commands and functionality.
- indentation: Python uses white-space indentation, rather than curly braces or keywords, to delimit blocks.
- int: An immutable integer of unlimited magnitude.
- interactive mode: When commands are read from a tty, the interpreter is said to be in interactive mode.
- interpret: Execute a program by translating it one line at a time.
- iterable: An object capable of returning its members one at a time.
- lambda: shorthand to create anonymous functions.
- list: Mutable array, can contain mixed types.
- list comprehension: A compact way to process all or part of the elements in a sequence and return a list with the results.
- literals: notations for constant values of some built-in types.
- map: map(function, iterable, …) Apply function to every item of iterable and return a list of the results.
- methods: A method is like a function, but it runs “on” an object.
- module: The basic unit of code reusability in Python. A block of code imported by some other code.
- object: Any data with state (attributes or value) and defined behavior (methods).
- object–oriented: allows users to manipulate data structures called objects in order to build and execute programs.
- pass: Needed to create an empty code block
- reduce: reduce(function, sequence) returns a single value constructed by calling the (binary) function on the first two items of the sequence, then on the result and the next item, and so on.
- run: this basically tells the computer to follow the commands in the code.
- set: Unordered set, contains no duplicates
- slice: Sub parts of sequences
- str: A character string: an immutable sequence of Unicode codepoints. Strings can include numbers, letters, and various symbols and be enclosed by either double or single quotes, although single quotes are more commonly used.
- statement: A statement is part of a suite (a “block” of code).
- try: Allows exceptions raised in its attached code block to be caught and handled by except clauses.
- tuple: Immutable, can contain mixed types.
- variables: Placeholder for texts and numbers. The equal sign (=) is used to assign values to variables.
- while: Executes a block of code as long as its condition is true.
- with: Encloses a code block within a context manager.
- yield: Returns a value from a generator function.